Android Mobile SDK 0.1.6
Document Version: 1.0.5 | zetacore:0.1.6
SDK Support & Compatibility
zeta-core
: android 7(API-24) and above
Install SDK
Install SDK from maven: https://mvnrepository.com/artifact/net.zetaglobal.app
- zeta-core
implementation(“net.zetaglobal.app:core:0.1.6”)
Mobile App Registration
Please follow the instructions outlined in the provided link to register your app with the ZMP platform: https://knowledgebase.zetaglobal.com/kb/mobile-app-registration
Initialize SDK
//ZetaClient should initialize inside the application class onCreate
//Create the configuration object
val config = ZTConfig(
clientSiteId = siteId,
clientSecret = clientSecret,
isLoggingEnabled = true,
optIn = true,
region = ZTRegion.US
)
//Initialize method
ZetaClient.initialize(this, config)
ZTPush.initConfig(
ZTNotificationConfig(
smallIcon = applicationInfo.icon,
color = Color.GREEN,
notificationChannel = ZTNotificationChannel(
id = "ApplicationTestChanne",
name = "Application Test Channe",
description = "Test Channel Description",
)
)
)
ZTConfig parameters
clientSiteId
: will be provided from ZMP console post successful app registrationisLoggingEnabled
: The value for enabling SDK logs, by default the parameter value will be disabledclientSecret
: will be provided from ZMP console post successful app registrationoptIn
: The value of enabling the SDK to track events and send data to the backend.region
: The SDK supports region configuration; the correct region should be referenced from ZTRegion class. Region cannot be interchanged for same ZMP Account.
ZTNotificationConfig parameters
smallIcon
: Set the small icon to use in the notification layouts.largeIcon
: Sets the large icon that is shown in the notification(This will be removed in version 1.0.0)color
: Accent color to be applied to the notification.enableAppLaunch
: Enable SDK launch the application, when notification actions are missing in payload(By default this flag will be true)notificationChannel
: Notification channel info. If this configuration is not provided, the SDK will create a DEFAULT channel
ZTNotificationChannel
parameters
id
: Channel idname
: Channel Namedescription
: Channel descriptionshowBadge
: Enable/Disable badge icon for the channel(By default this flag will be true).autoCancel
: Setting this flag will make it so the notification is automatically canceled when the user clicks it in the panel(By default this flag will be true)notificationSound
: Resource id for the explicit sound file(Optional field)
How to initialize the ZetaClient?
The ZetaClient
should be initialized inside the onCreate
method of your application. During this initialization, only the site ID is a mandatory field, and by default, logging will be disabled.
For the SDK to communicate with the server, clientSecret is required. The clientSecret can be provided either during the SDK initialization or through a late setClientSecret
method.
ZetaClient.setClientSecret(context = applicationContext, clientSecret = clientSecretKey)
How get the BSIN from the SDK?
The SDK exposes a method for retrieving BSIN changes. This callback lambda will be triggered whenever a BSIN change occurs from the SDK side.
ZetaClient.setOnBsinChange { bsin ->}
To get the cached BSIN, use ZetaClient.user.getCachedBsin()
Tracking Opt-in and Opt-out
When the application does not require the SDK to track events and user properties, it can utilize the opt-out feature. The application sends the opt-out configuration as part of its initialization or can call a separate opt-out method. If the application is using SDK-exposed methods for opting in or out, the same status should be sent through the SDK initialization configuration during the next launch. Otherwise, the configuration value may overwrite the opt-in/out status. Once the SDK receives the opt-out request, it will immediately stop all communication with the back end, and any data cached in the database will also be cleared. Until the application opts back in, the SDK will neither collect data from the application nor send data to the server. The application can opt in or out at any time during its lifecycle. After opting out, if the application opts back in, the SDK will consider it a fresh launch.
Tracking opt-out
Tracking of SDK can be disabled in two ways:
Pass opt-in as false during initialization
ZTConfig(
clientSiteId = siteId,
isLoggingEnabled = true,
optIn = optInStatus, //This value can be true or false
region = ZTRegion.EU
)
Call stop tracking method
ZetaClient.optOutFromTracking(context = context)
Here context is the Application context.
Tracking opt-in
To start tracking again, you can pass optIn as true in the configuration object when calling ZetaClient.initialize(this, config)
, or you can call
ZetaClient.optInForTracking(uid = uid)
.
_uid_
: At the time of opt-in, the uid field is optional for identifying the user from the SDK's perspective. If the application does not provide a user ID, the SDK will consider the user as an anonymous user.
User Identification
Update User Properties
The ZTUserManager
instance is exposed from the ZetaClient
for performing user operations. The client app can update the user data using the builder pattern or by creating a ZTUser
object at the app level and calling the setUser
method to pass the data to the SDK.
ZetaClient.user.builder()
.setName(name = name.value)
.setFirstName(firstName.value)
.setLastName(lastName.value)
.setSource(source.value)
.setEmail(ZTUserEmail(email = email.value, additionalInfo = emailAdditionalInfo.value))
.setUid(userid.value).setSignedUpAt(signedUp.value).setPhone(
ZTUserPhone(
phone = phoneContact.value, additionalInfo = phoneAdditionalInfo.value
)
).submit()
or
val user= ZTUser(name = "John Wick")
ZetaClient.user.setUser(user)
For contact information (email, phone number), the client app can also pass the additional info to the SDK.
//ZTContactAdditionalInfo
val contactAdditionalInfo = ZTContactAdditionalInfo(
preferences = preferencesList,
subscriptionStatus = subscriptionStatus.value,
inactivityReason = contactInactivityReason.value,
signedUpAt = contactSignedUpAt.value,
doubleOptInStatus = contactDoubleOptInStatus.value,
phoneType = contactPhoneType.value,
lastClicked = contactLastClicked.value,
lastSent = contactLastSent.value,
lastOpened = contactLastOpened.value,
contactProperties = contactExtraPropertyMap.toMap()
)
preferences: List<String>, the defaule value will be "standard"
subscriptionStatus:ZTContactSubscriptionStatus, This field value can be, NEW, ACTIVE,INACTIVE
contactProperties: Additioncal contact properties can through this map
- preference of contact defaults to
standard
but other preferences can be added/customized within the settings menu in ZMP. Only those defined values can be passed in preference double_opt_in_status
optional field to track double opt ins - default value is null, but will accept single or double valuesphone_type
optional field for phone contact types. default value is null, accepts mobile or landline values
Date Time Format Specification
All date and time values should be in ISO 8601 format. The SDK exposes an extension method for converting millisecond timestamps to ISO 8601 format. The method usage example.
System.currentTimeMillis().formatDateToIso()
How to Start the User identity Session?
The SDK supports tracking user session-specific properties and events. Setting the uid
property will facilitate user identity session tracking.
- Using builder pattern
ZetaClient.user.builder().setUid(userName.value).submit()
- Using the User Object
val user = ZTUser(uid = "userId")
ZetaClient.user.setUser(user)
How to Clear the User identity Session?
The user manager's clear
method will remove the stored user identity session
ZetaClient.user.clear()
How to pass appid, gaid(advertisementId) and firebase device token?**
- App Set Id : To set the app ID, use the following method:
ZetaClient.user.setAppSetId(id)
- Google AdvertisementId(GAID) : To set the Google Advertisement ID, use the following method:
ZetaClient.user.setAdvertisementId(id!!)
- Firebase device token : To set the Firebase device token, use the following method:
ZetaClient.user.updatePushToken(token)
Location Tracking
location
: This event can be called by passing the location coordinates along foreground/background state. The foreground/background state indicates whether the location data is collected when the app is in the foreground or background.The provided location will be cached in memory, and for subsequent events, the location details
will be added as a property.
ZetaClient.user.trackLocation(
latitude = 36.778259,
longitude = -119.417931,
isForeground = true
)
Firebase Registration
The application needs to handle Firebase registration and must keep the google-services.json
file inside the project/app folder.
Event Tracking
Auto tracked events
app_installed
: This event is generated only once when the app is launched for the first time.app_opened
: This event is generated whenever the app transitions from the background to the foreground.app_closed
: This event is generated whenever the app transitions from the foreground to the background.
Defined events
Screen Name Tracking
trackScreenName
: This event is used for tracking user screen navigation. The provided screen name will be cached, and for subsequent events, the screen_name
will be added as a property.
screenName
: Application active screen namedeeplink
: An optional field for specifying that this screen is opened through a deeplink URL.properties
: An optional field for specifying additional properties.
ZetaClient.event.trackScreenName(screenName = "screenName", deeplink = "deeplink url", properties = optionalProperties)
Custom Events
The SDK accepts custom events. To send custom events, the application needs to pass the event name and properties to the SDK.
eventName
: A meaningful name that describes the event.properties
: A map of event properties, where the property values should be JSON encodable and decodable. If any property value is not JSON encodable, the entire event will be discarded.
ZetaClient.event.send(eventName = "eventName", properties = eventProperties)
Push Notification
To set up push notifications, the application needs to manage Firebase registration and ensure that the google-services.json
file is placed in the correct path (project/app).
The push notification service will not work without Google Play services, so the application must ensure that the device has Google Play services installed.
For Android 13 and above, user permission is required to show notifications, and the application must handle this permission appropriately.
Firebase dependency
Application needs to add the firebase dependency inside app/build.gradle file
implementation("com.google.firebase:firebase-messaging:24.1.0")
Before using the library ensure we are using the latest version.
How to configure push service?
The SDK includes a built-in push service that needs to be registered in the application manifest file. Add the following snippet to your AndroidManifest.xml
:
<service
android:name="net.zetaglobal.app.zetacore.push.ZTPushService"
android:exported="false">
<intent-filter>
<action android:name="com.google.firebase.MESSAGING_EVENT" />
</intent-filter>
</service>
Configure the push notification and channel info:
ZTPush.setNotificationConfig(
ZTNotificationConfig(
smallIcon = applicationInfo.icon,
color = Color.GREEN,
enableAppLaunch = true,
notificationChannel = ZTNotificationChannel(
id = "ApplicationTestChannel",
name = "Application Test Channel",
description = "Test Channel Description",
showBadge = true,
autoCancel = true,
notificationSound = R.raw.test_ring_tone_1
)
)
)
ZTNotificationConfig parameters
smallIcon
: Set the small icon to use in the notification layouts.color
: Accent color to be applied to the notification.enableAppLaunch
: Enable SDK launch the application when user tap on it, when notification actions are missing in payload(By default this flag will be true).notificationChannel
: Notification channel info. If this configuration is not provided, the SDK will create a DEFAULT channel.
ZTNotificationChannel parameters
id
: Channel idname
: Channel Namedescription
: Channel descriptionshowBadge
: Enable/Disable badge icon for the channel(By default this flag will be true).autoCancel
: Setting this flag will make it so the notification is automatically canceled when the user clicks it in the panel(By default this flag will be true)notificationSound
: Resource id for the explicit sound file(Optional field)
Setting Up Push Notification Deep Linking
When a user taps on the notification, the intent will be delivered to the application. In the AndroidManifest.xml
, the deep link URL must be registered to launch the activity. For example:
<intent-filter>
<action android:name="android.intent.action.VIEW" />
<category android:name="android.intent.category.DEFAULT" />
<category android:name="android.intent.category.BROWSABLE" />
<data android:scheme="com.zeta.android.demo" />
<data android:host="home" />
</intent-filter>
Tracking Foreground Notification Deep Link Clicks
The notification payload will be delivered to the registered activity through the intent. The deep link URI value can be retrieved from intent.data
. To process the activity intent, pass it to the SDK. If the intent is valid, the SDK will return a valid notification info object, which can then be used for tracking. For example:
private fun handleIntent(intent: Intent?) {
val data = intent?.data
data?.let { uri ->
appViewModel.setDeepLinkUri(uri)
}
intent?.let {
val notificationInfo = ZTPush.getNotificationInfo(intent)
notificationInfo?.let {
ZTPush.trackEvent(notificationInfo)
ZTPush.removeNotification(
context = applicationContext,
notificationInfo.localNotificationId
)
}
intent.data = null, // Clear the data after processing
}
}
Setting Up Push Notification Action Callback
Foreground Action
When a user taps on a notification action button while in the foreground, the respective action will be delivered to the application. The application should register the specified action in the activity's configuration section in the AndroidManifest.xml
. For example:
<intent-filter>
<action android:name="com.zeta.demoapp.open_notification" />
<category android:name="android.intent.category.DEFAULT" />
</intent-filter>
Tracking Foreground Action Button Clicks
The notification payload will be delivered to the registered activity through the intent. To process the activity intent, pass it to the SDK. If the intent is valid, the SDK will return a valid notification info object, which can then be used for tracking. For example:
val notificationInfo = ZTPush.getNotificationInfo(intent)
notificationInfo?.let {
ZTPush.trackEvent(notificationInfo)
//Removing notifcation from the system tray.
ZTPush.removeNotification(
context = applicationContext,
notificationInfo.localNotificationId
)
}
Background Action button
When a user taps on a background action button, the SDK will pass the callback to the method below. Once this method is invoked, the application can process it. For this action, the SDK will automatically create the event:
ZTPush.setActionBackgroundCallback { data ->
//Process the noticiation background action click
}
Custom Push Service
If you want to use your own custom PushService
, implement the following method to send the device token to the server in the onNewToken
method:
override fun onNewToken(token: String) {
super.onNewToken(token)
ZetaClient.user.updatePushToken(token)
}
Processing Push Notifications
To process the push notifications, use the following method:
ZTPush.handleMessage(
context = applicationContext,
remoteMessage = message
)
If the above method returns true
, the SDK is able to process the notification. If it returns false
, the application needs to handle the notification accordingly.
Delivery Status of Push Notifications
The SDK automatically captures the delivery status of push notifications in both cases(in service/Custom Service)
How to handle non zeta notification?
For handling unfamiliar notifications, the application can register a callback lambda method in ZTPush.
ZTPush.setNonZtNotificationCallback {remoteMessage->
Log.d("ZTDemoApp", "Unknown notification received:$remoteMessage")
}
Types of Notifications
The Android push SDK supports two primary types of notifications:
Text Type Notification
Text notifications consist of a notification message along with text content. If any action buttons are specified in the payload, they will also appear in the notification.
Anatomy of Text Notification:
-
Left Side: Displays the app icon or a specified icon from the payload. If an icon parameter is included in the payload, it should be bundled in the Android drawable folder. If not, the default app icon will be shown.
-
Title: The title displayed is derived from the payload.
-
Content: The body text shown is also taken from the payload.
-
Action Buttons: Any action buttons specified in the payload will be displayed as part of the notification actions.
Image Type Notification
Image type notifications function similarly to text notifications but additionally support an image. The image URL must be specified in the payload and should be publicly accessible.
Anatomy of Image Notification:
Left Side: Displays the app icon or a specified icon from the payload. If an icon parameter is included in the payload, it should be bundled in the Android drawable folder. If not, the default app icon will be shown.
Title: The title displayed is derived from the payload.
Content: The body text shown is also taken from the payload.
Image Icon: The right side of the notification displays the actual image as an icon. When the notification is expanded, a larger version of the image will appear, and the right icon will disappear. If the SDK fails to load the image, only the title and content will be shown. For optimal user experience, it is recommended that images maintain a 2:1 aspect ratio. Additionally, if there is any text in the image, it should be centered to avoid being cut off when displayed in the notification.
Action Buttons: Any action buttons specified in the payload will be displayed as part of the notification actions.
In App Message
In-app push messaging allows marketers to queue up toaster-style messages within their app that will display when users open the app. Opt-in/consent is not required, so deliverability is near 100% of app install base. This message type is great for highly contextual, low urgency messages.
In-app messages are displayed within mobile app without requiring any additional permissions.
The SDK uses a polling mechanism to fetch in-app messages. Once a message is received, the SDK evaluates the app state to determine if it should display the in-app message. If conditions are met, an in-app message dialog will be shown.
To monitor in-app messages, the SDK provides an in-app message lifecycle callback that can be configured from the application
Start and Stop In App Messages
In App message is enabled by default, but if the app needs to stop in app message the app can call:
ZetaClient.inApp.stop()
To restart In App message again, the app can call:
ZetaClient.inApp.start()
Note: The SDK does not persist the stop state, the application must manage this state. By default, the SDK will start displaying in-app messages on app launch.
In App Message Callbacks
The SDK provides lifecycle callbacks for in-app messages that can be configured within the application. The callback methods include:
- fun willAppear(dataModel: ZTInAppMessage): Invoked when the SDK is about to show the message.
- fun didAppear(dataModel: ZTInAppMessage): Invoked after the message has appeared.
- fun onClicked(dataModel: ZTInAppMessage, deeplinkUrl: String?): Invoked when a user clicks on the message.
- fun onDismissed(dataModel: ZTInAppMessage): Invoked when the message is dismissed.
ZetaClient.inApp.setCallBack(object : ZTInAppMessageLifeCycle{
override fun willAppear(dataModel: ZTInAppMessage) {
}
override fun didAppear(dataModel: ZTInAppMessage) {
}
override fun onClicked(dataModel: ZTInAppMessage, deeplinkUrl: String?) {
}
override fun onDismissed(dataModel: ZTInAppMessage) {
}
})
How to Create an In-App Message
- Access the ZMP Portal
- Log in to the ZMP portal.
- Configure the Campaign
- Navigate to the campaign window.
- Select the channel as 'Mobile InApp Push'.
- Compose the Message
- Click on the In-App Messaging Setup content area to begin composing your message.
- Message Composition Steps
- Title: Provide a title for your message.
- Body: Provide the body of your message
- OnClick Options: Choose one of the following actions for when a user taps on the message:
- Deep Link: When the user taps on the message, the SDK will deliver the deep link URL to the application through a delegate method. The application must handle internal redirection.
- Dismiss: No action will be performed when the message is clicked.
- Web Link: The specified URL will open in a browser view when tapped.
- In-App Web View: The specified URL will open inside the SDK in SFSafariViewController
- External Browser: The specified URL will open in the device's default browser.
- In-App Message Image (Optional): An image can be specified through available options.
- Finalize Your Message
- After composing your message, click on the Done button.
- Activate the Campaign
- Activate your campaign from the portal to start displaying in-app messages.
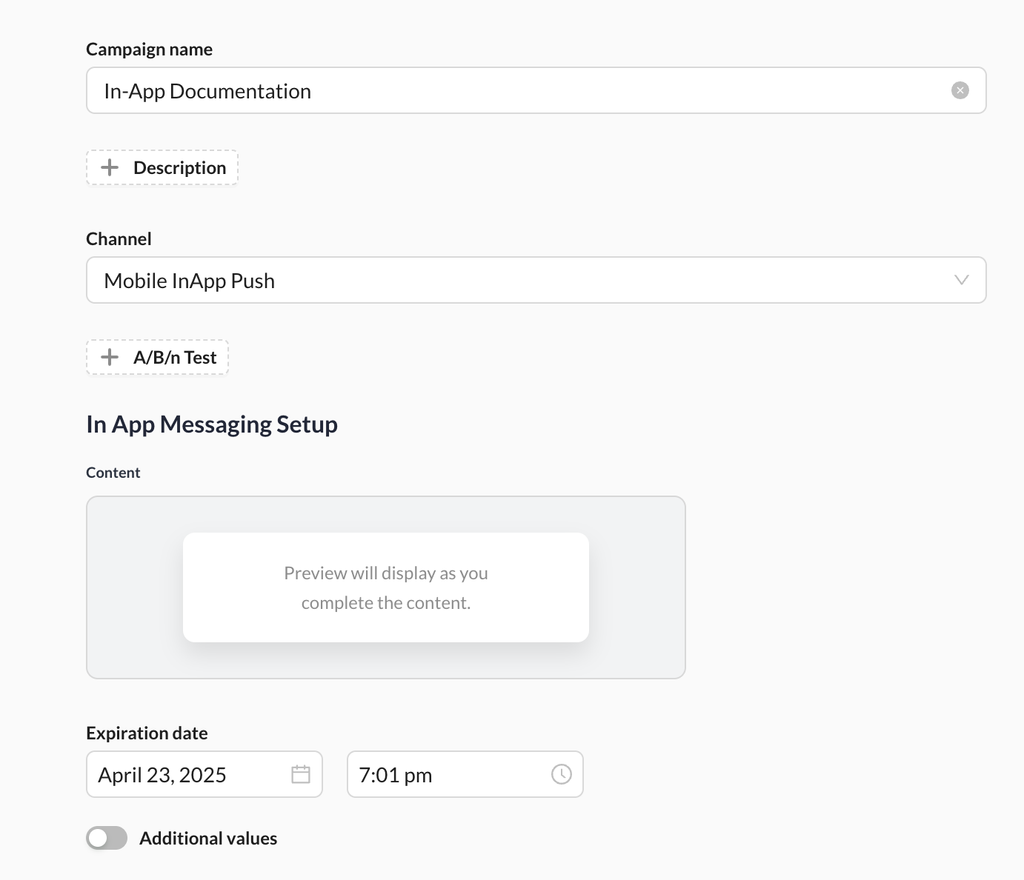
InApp Setup view
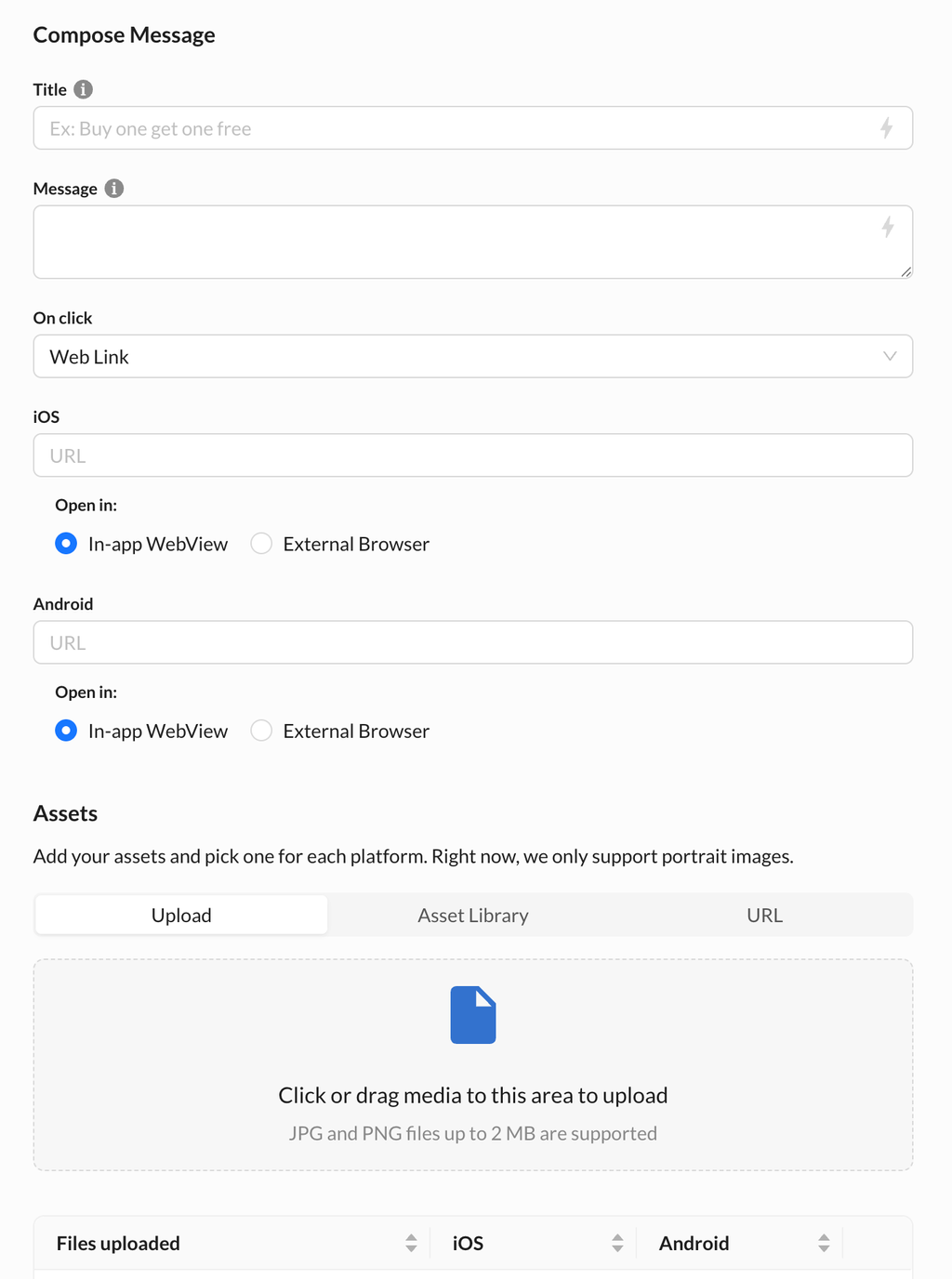
InApp Compose View
Updated 3 days ago